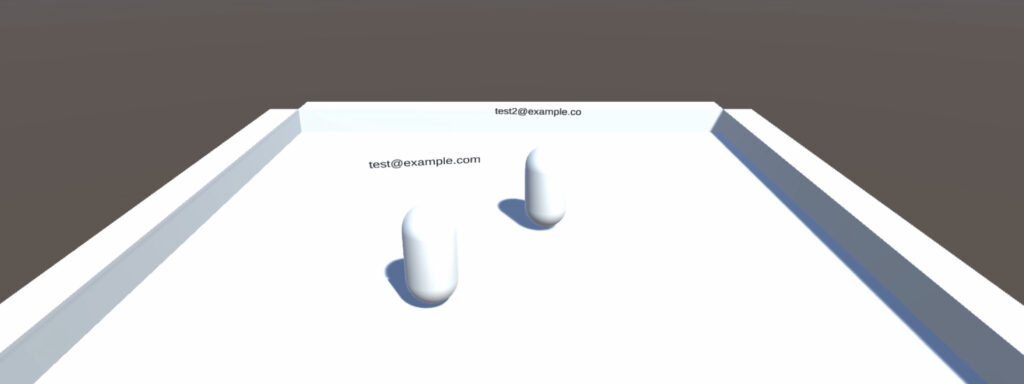
How are Massively Multiplayer Online RPGs built? What sort of infrastructure do they need? How can I make an online multiplayer game myself?
These are all valid questions, and ones we’ll be covering here!
For context, we’ve worked on a lot of different video game projects here at Noble Steed Games, including online multiplayer games, but MMOs have been something we’ve always wanted to explored! So we started a video series about making one from scratch! This blog exists as a full transcription of the full video for those who prefer text-based resources. Enjoy!
In this first part, our goal will be to take a simple little game where capsules move around, and make it networked. This way, two different players can sign up, join the game, and do a little dance.
Network infrastructure for our MMO
Modern multiplayer games are quite complex, and are built from lots of systems, but the ones we’re going to focus on for this video are:
- Game client: The thing that the player downloads to play the game
- Game server: Hosts the ‘rooms’ that the players will be joining, and manages real-time networking between players
- Game backend: Stores more permanent data and information (like authentication information, or player inventories).
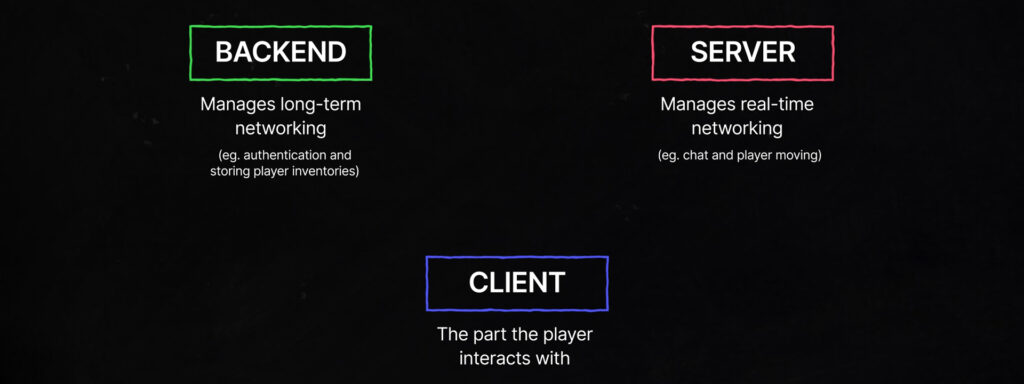
It’s worth noting that backend and server can sometimes both be referred to as the ‘Server’, but to keep things simple, we’re splitting their definitions as ‘Server’ and ‘Backend’. We’ll start with the ‘Server’.
Rest APIs are simple web services that receive requests and respond to them. For this project, we’ve decided to build the backend using DotNET. This is a pretty common stack for Rest APIs, which is most of what we’ll be using it for.
It’s worth noting that there are a lot of decisions that we’ll be making for this project which depend on its specific purposes. We’ve decided to use Dotnet for this project because it’s in C#. It is used a lot for simple REST API type web-apps, and is pretty easy to set up. We just need a few simple pieces at the moment: a database which stores Users, and the endpoints which allow users to sign up and log in. In Unity, we write some simple wrappers to interface with these endpoints, and we’ll be able to log in.
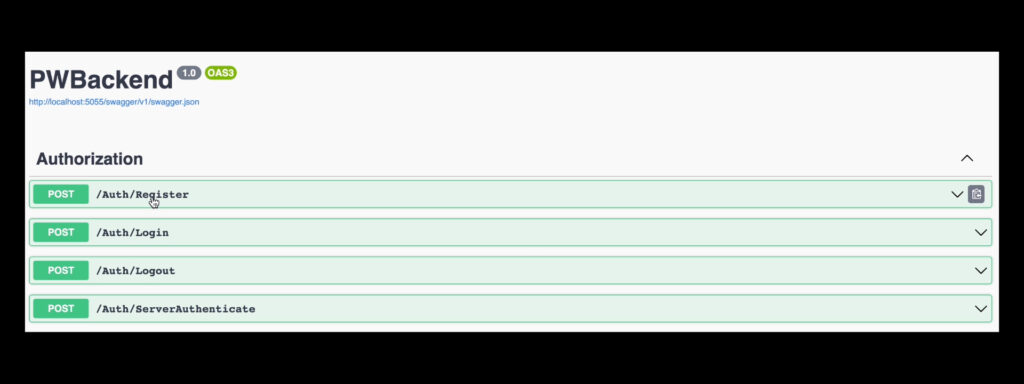
The other thing that the Backend is going to be used for is server orchestration. Right now, our Backend is set up so that it spawns a single Server that everybody connects to, but in the future, we’ll probably want to have a whole system of different servers, depending on things like player count and regions. This will come in the future, but for right now we just have the Backend spawning a single server. It’s a little janky, but will do for this point.
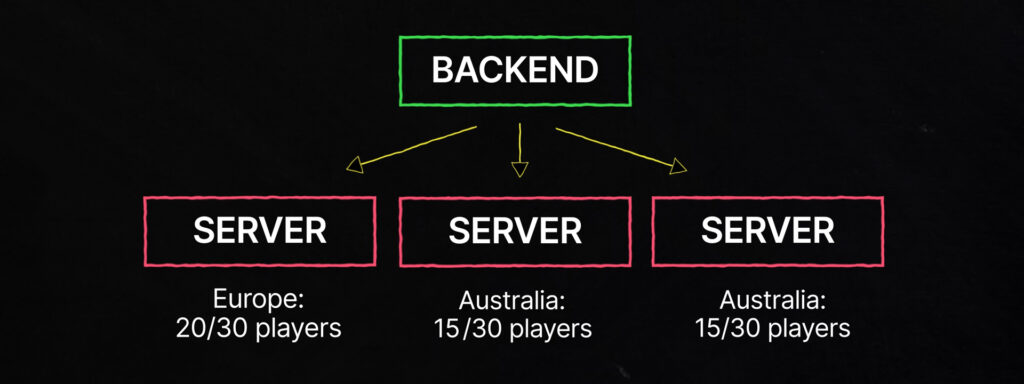
Getting our game Networked with Photon Fusion
Heading over to the actual game, our next step is going to be getting this networked. For this, we’ll be using Photon Fusion. We’ve used it for other projects, and our game doesn’t involve any networked physics, in which using a different engine might be more fitting.
With that in mind, we can follow the simple Fusion tutorials to get a basic game up and running. First, we’ll set this up as a simple shared peer-to-peer networked experience, create our networked input, add a networked transform, and we’re good! Then, it’s not too complex to change it to being a server-hosted experience with clients connecting.
Once we’ve got the basics of each of our three pieces up and running, the next step is to connect them a bit more actively. Before we can do that though, we should talk about trust.
Backend Security
Currently, our client sends their login information to the backend. This confirms they are who they say they are, and gives them all their info. Then, they connect to the server. However – there’s an issue here. The client can’t be responsible for telling the server who they are, because they could lie. If a client says that they’re an admin, or some other player, the server shouldn’t believe them. The general principle for networked games like this is that the backend and the server should never trust the client. So, how do we get around this? There are a few different answers of different complexity, but we’re going to go with a fairly simple system.
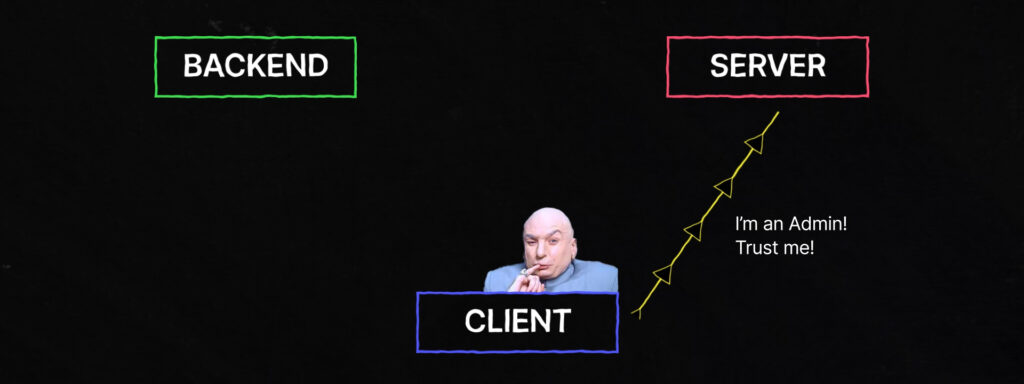
First, the client logs in to the backend, with their username and password. Assuming that they’re correct, the backend knows who that client is, and allows them to access their information. Next, the client connects to the server, and gets a unique ID there – the server doesn’t know yet who this ID belongs to, but the server knows this client matches this specific ID. Next, the client passes that ID to the backend, who confirms that it is coming from the person they’ve already authenticated and tells the server “Hey – this ID belongs to this specific user”. That way, the server also knows that that ID belongs to that user, without ever trusting the user themselves. This isn’t a perfect solution, a more advanced one using proper tokens is probably what we’ll implement later, but it’ll serve our purposes for right now.
The last thing to do is make the players distinguishable, in this case via their emails. Now, both users can log in, see the email address of the other user (which has been authenticated by the backend and provided to the server), and can run around and interact.
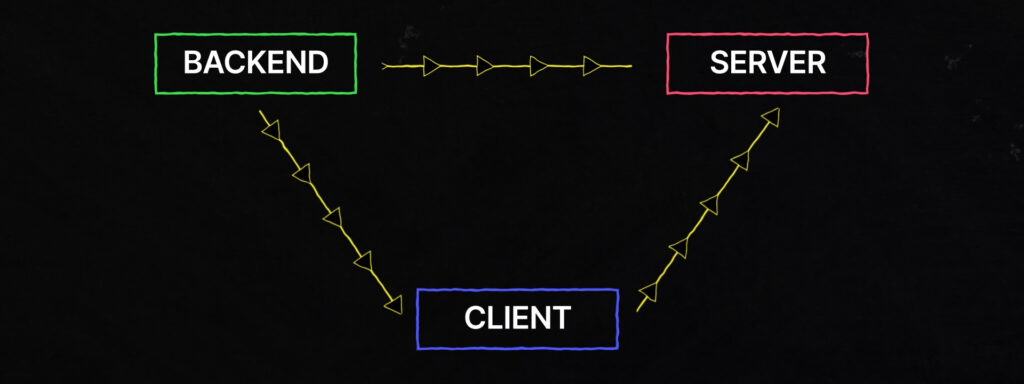
And that’s how we’ve set up our simple network infrastructure! We’ve got a long way to go before this is a real MMORPG, but these are the basic pieces that get it all working. Next time – we’ll make this look a bit more like an MMO with actual characters moving around and chatting to each other.
We hope you enjoyed reading this! Have a question or want to chat more about game development? Reach out to us!
Other places you can find us:
- Our other game development resources
- Join our Discord server